🔍 How to Implement Search in a Backend Web Application
Implementing an effective search feature is essential for any modern web application. In this guide, we explore how to build search functionality in a backend system—from basic SQL queries to advanced full-text search and scalable search engines like Elasticsearch.
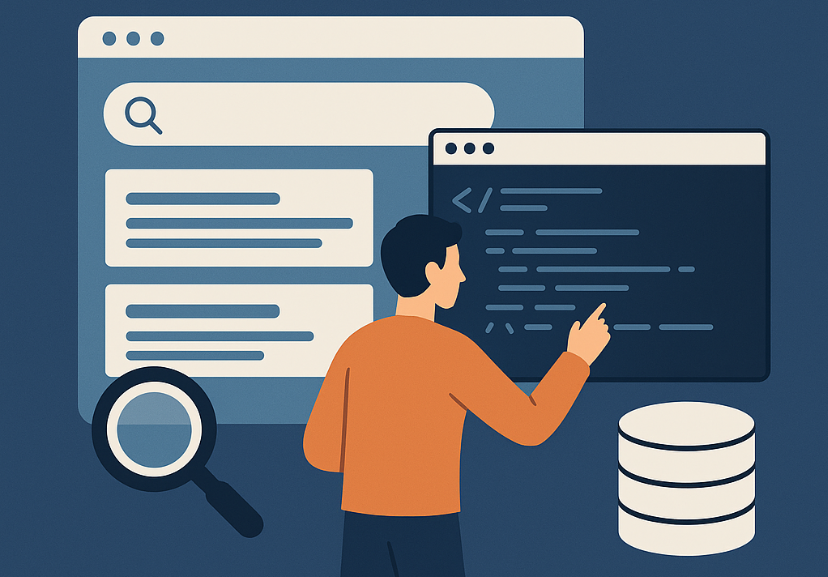
Whether you're building an e-commerce platform, a blog, or a knowledge base, search is one of the most essential features users expect. In this post, we’ll walk through how to implement search in a backend web application, focusing on both simple and scalable approaches.
💡 Why Search Matters
Users want to find relevant content quickly. A good search feature improves:
- User experience
- Retention
- Conversion rates (in e-commerce and SaaS platforms)
Let’s dive into how to build it.
🧱 1. Choose Your Stack
Assuming you already have a backend built with something like Django, FastAPI, Express.js, or Laravel, you’ll be working with:
- Database (e.g., PostgreSQL, MySQL, MongoDB)
- ORM (like SQLAlchemy, Django ORM, Prisma)
- Optional: Search engine (e.g., Elasticsearch, Typesense, Meilisearch)
🔎 2. Basic Search Using SQL Queries
This is the most straightforward approach using the database’s LIKE
operator or full-text indexing.
Example (PostgreSQL + SQLAlchemy):
from sqlalchemy import or_
def search_articles(session, keyword):
return session.query(Article).filter(
or_(
Article.title.ilike(f"%{keyword}%"),
Article.content.ilike(f"%{keyword}%")
)
).all()
Pros:
- Easy to implement
- No extra tools required
Cons:
- Limited ranking/relevance
- Slow on large datasets
🧠 3. Full-Text Search (FTS)
Most relational databases support full-text search, offering better relevance scoring.
PostgreSQL Example:
SELECT *
FROM articles
WHERE to_tsvector('english', title || ' ' || content)
@@ plainto_tsquery('english', 'python backend search');
Django ORM with SearchVector
:
from django.contrib.postgres.search import SearchVector
Article.objects.annotate(
search=SearchVector('title', 'content')
).filter(search='python backend search')
Pros:
- Better ranking and relevance
- Still simple and in-database
Cons:
- Requires schema tuning
- Doesn’t scale as well as dedicated engines
🚀 4. Scalable Search with External Engines
If you have more advanced requirements (synonyms, typo-tolerance, relevance ranking), integrate a search engine:
Popular Options:
- Elasticsearch – powerful, complex, scalable
- Meilisearch – lightweight, fast, dev-friendly
- Typesense – great balance of performance and ease-of-use
Example: Search API with FastAPI + Meilisearch
from fastapi import FastAPI, Query
import meilisearch
app = FastAPI()
client = meilisearch.Client("http://localhost:7700", "masterKey")
@app.get("/search")
def search(q: str = Query(...)):
results = client.index("articles").search(q)
return results
Syncing Your Data
Use background jobs (Celery, RQ, etc.) or signals/hooks to sync data from your database to the search index.
Pros:
- Great UX (autocomplete, typo handling, filters)
- High performance at scale
Cons:
- Extra infrastructure to manage
- Data syncing complexity
🛠 5. API Design Tips
Your search API should:
- Use
GET /search?q=term
for simple queries - Return paginated results
- Allow filters/sorting if needed
- Normalize and sanitize input
{
"query": "python",
"results": [
{"id": 1, "title": "Intro to Python", "snippet": "..."},
{"id": 2, "title": "Python for Web", "snippet": "..."}
],
"page": 1,
"total": 42
}
🔐 6. Security & Optimization
- Rate limit public search endpoints
- Escape input for SQL injection prevention
- Index frequently queried fields
- Use caching for common queries
🧩 7. Bonus Features to Consider
- Highlighting matched terms
- Autocomplete or search suggestions
- Faceted filters (categories, tags)
- Search analytics
✅ Conclusion
Implementing search starts simply with SQL and scales with full-text indexing or external search engines. Choose based on your project size, complexity, and performance needs.
TL;DR
Method | Best For | Tools |
---|---|---|
SQL LIKE |
Very small datasets | Native SQL |
Full-Text (FTS) | Medium complexity | PostgreSQL, Django |
External Search | Large-scale, typo-tolerant | Meilisearch, Elasticsearch |